Building modern apps can seem overwhelming with the many tools and technologies available. However, having the right tools can make a huge difference in the development process, helping developers work faster and more efficiently.
Whether you’re making a mobile application, a web application, or a desktop application, there are essential tools that can improve your workflow. This article will cover some must-have developer tools for building modern apps and explain how they can help you.
1. Code Editors and IDEs (Integrated Development Environments)
The foundation of any development work is the code editor or Integrated Development Environment (IDE) you use. A good code editor is essential for writing and editing your app’s code efficiently.
Visual Studio Code (VS Code)
Visual Studio Code is a free, open-source code editor developed by Microsoft that supports a variety of programming languages, offers a rich set of extensions, and has features like IntelliSense, debugging, and version control.
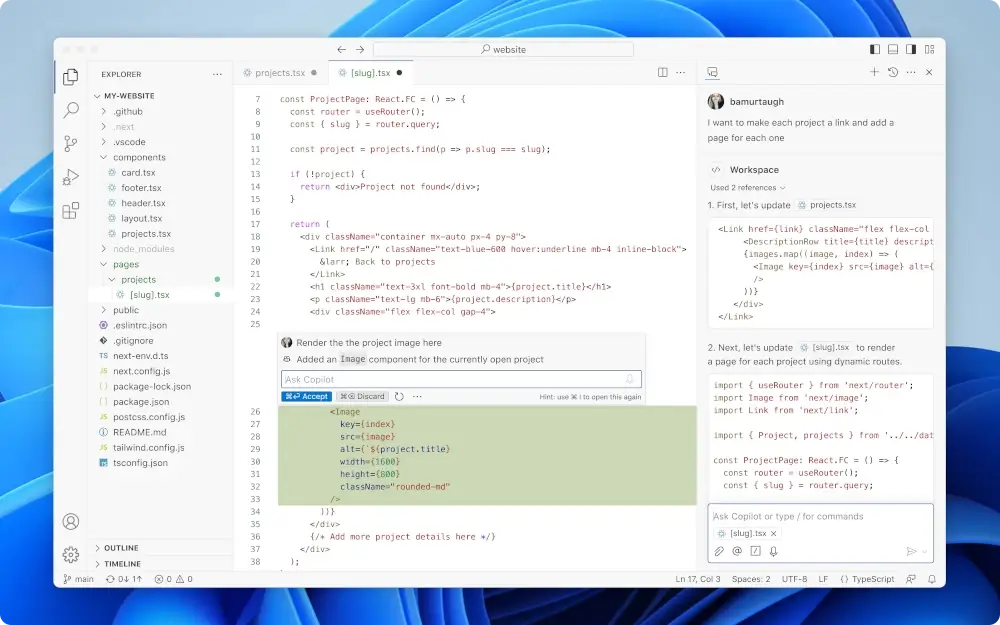
JetBrains IntelliJ IDEA
IntelliJ IDEA is a powerful IDE that’s especially good for Java development, though it supports many other languages and comes with smart code suggestions and easy refactoring tools.
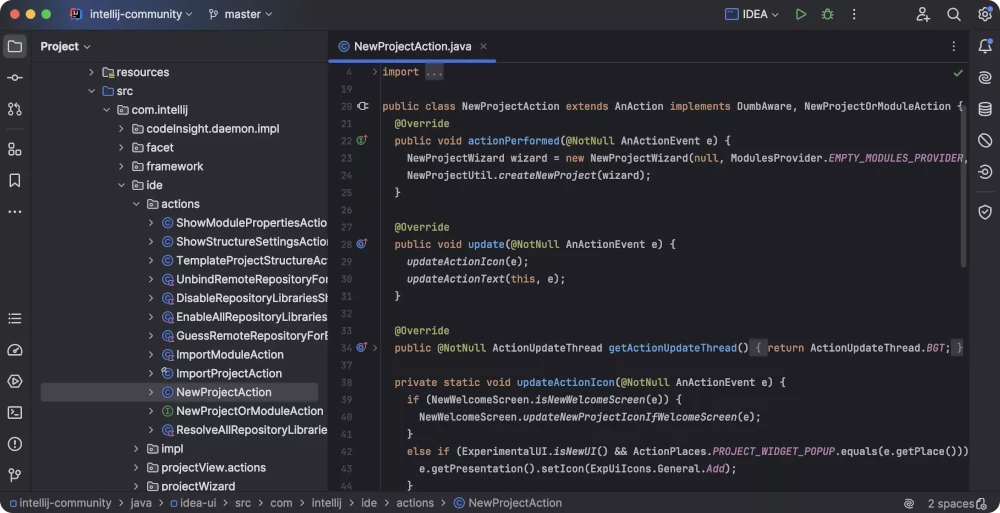
Sublime Text
Sublime Text is a lightweight code editor with a clean interface, ideal for quick edits or smaller projects, that also supports extensions and customizable features.
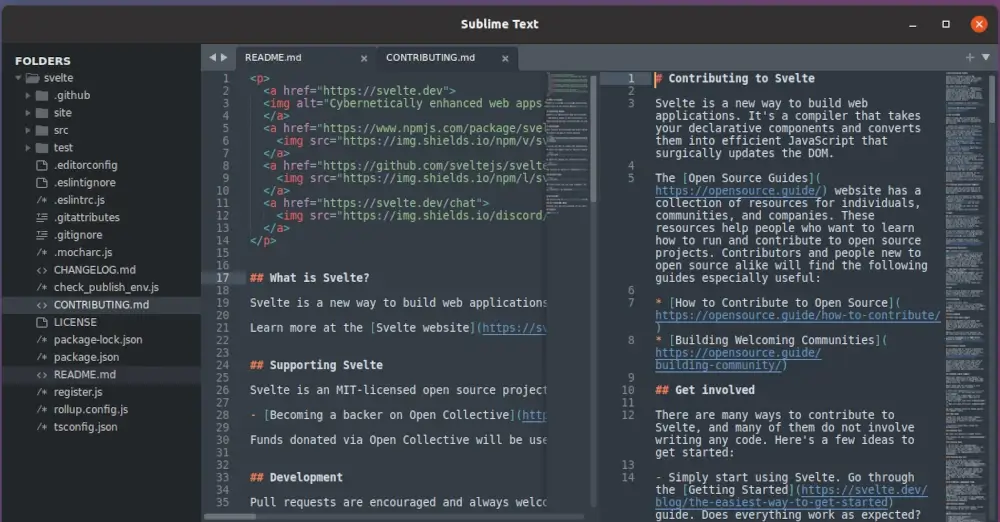
Vim Editor
Vim, short for “Vi Improved“, is a powerful, open-source text editor designed for both command-line and graphical interfaces.
It offers advanced capabilities which include syntax highlighting, macros, and support for numerous programming languages, making it suitable for a wide range of development tasks.
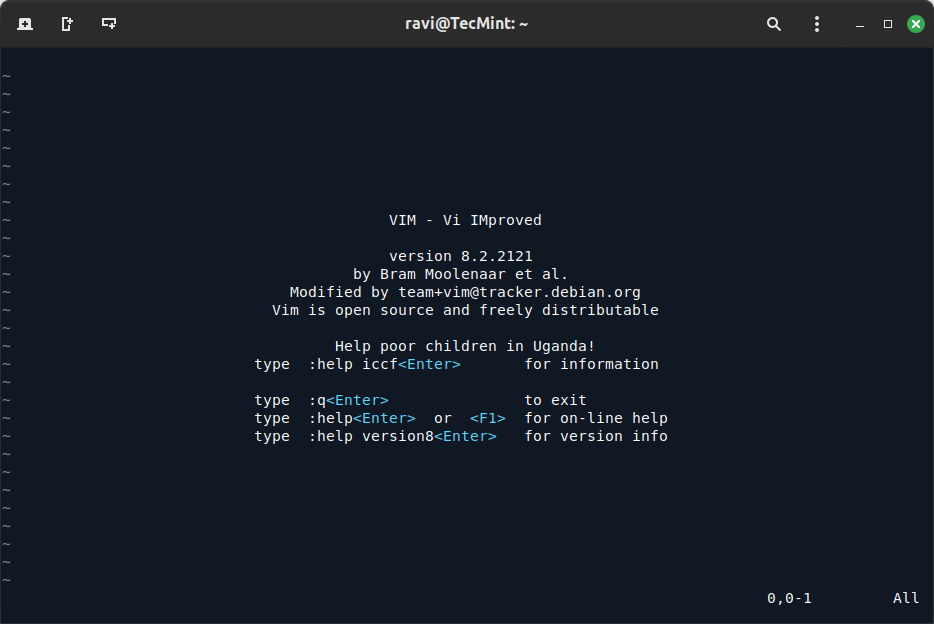
A code editor or IDE should be chosen based on your app’s development needs. For example, if you’re working with JavaScript or TypeScript, VS Code is an excellent choice because it supports these languages well.
2. Version Control Tools
Version control is crucial for tracking changes to your code, collaborating with other developers, and managing different versions of your app.
Git
Git is the most popular version control system used by developers worldwide, which helps you track changes in your code and share it with others.
Git allows you to go back to earlier versions of your app and resolve conflicts when multiple developers work on the same code.
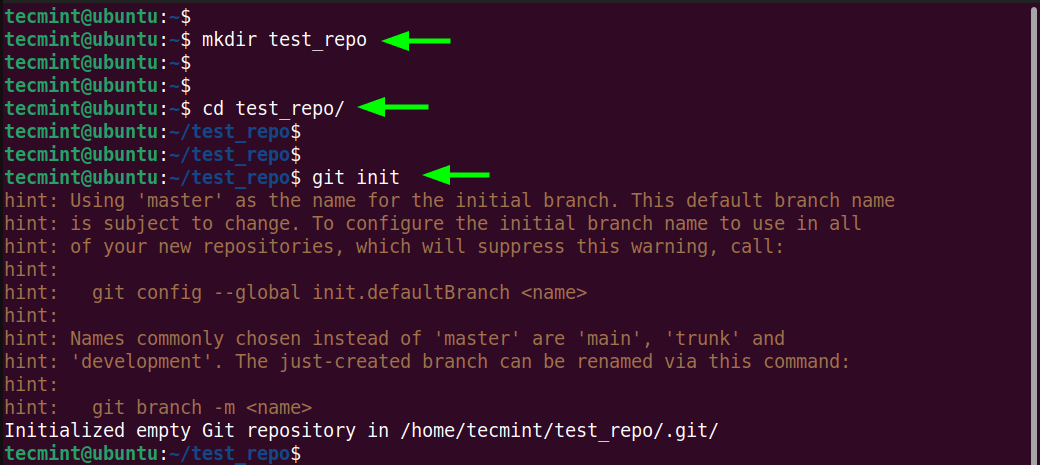
GitHub
GitHub is a platform that hosts Git repositories and offers features for collaboration, code reviews, and issue tracking. It’s ideal for open-source projects and team-based development.
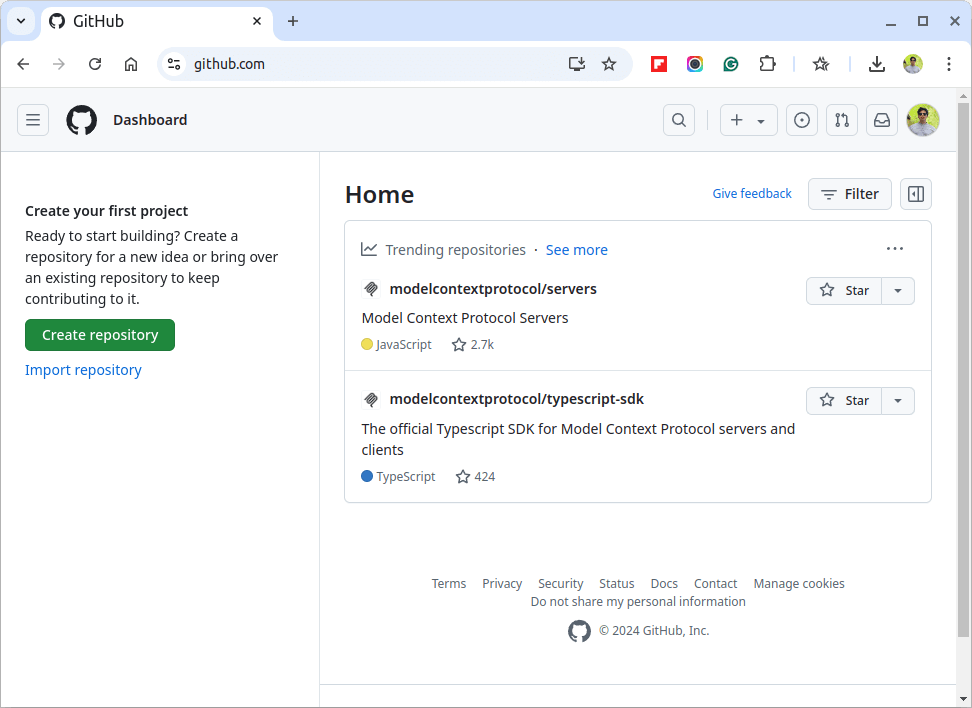
GitLab
GitLab is similar to GitHub but offers a Git repository platform with additional DevOps tools like CI/CD (Continuous Integration and Continuous Deployment) pipelines.
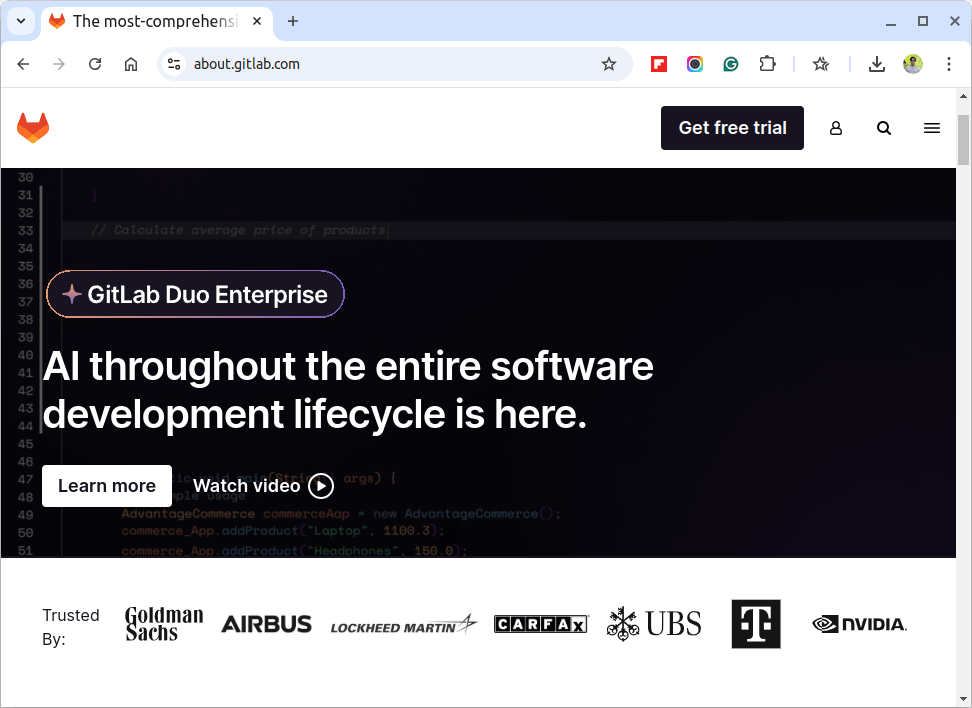
Bitbucket
Bitbucket is a Git repository management tool with a focus on team collaboration, which is especially popular for private repositories.
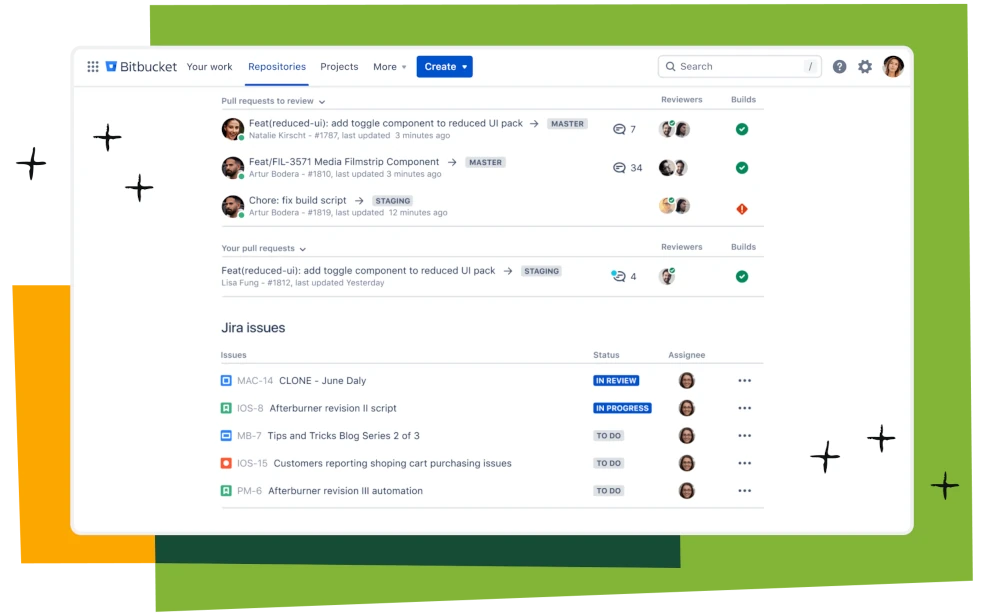
Version control helps you keep track of your code changes and collaborate with other developers without overwriting each other’s work. Learning Git is essential for any developer.
3. Package Managers
Managing dependencies is one of the key challenges in app development and package managers help you automate the process of installing, updating, and managing third-party libraries or frameworks your app depends on.
npm (Node Package Manager)
npm is the default package manager for Node.js
that will help you manage dependencies and install packages easily when you are working with JavaScript or building web apps.
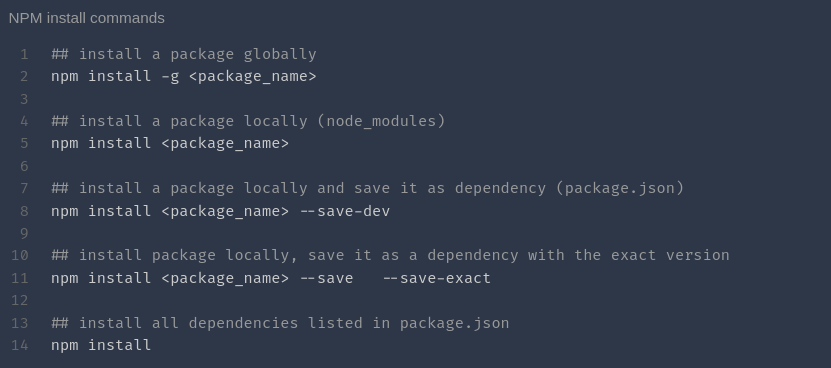
Yarn
Yarn is a faster alternative to npm that also helps manage dependencies for JavaScript projects. Yarn has built-in caching for faster installs and uses a lock file to ensure consistent package versions across different machines.
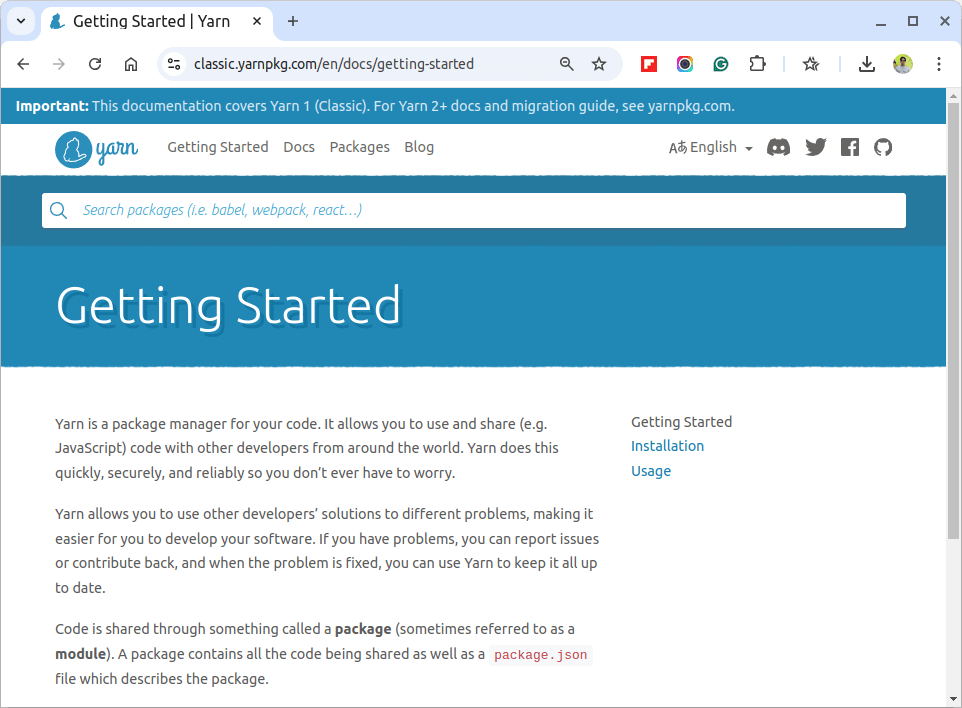
Homebrew
Homebrew is a package manager for macOS (and Linux) that allows you to install command-line tools and software easily.
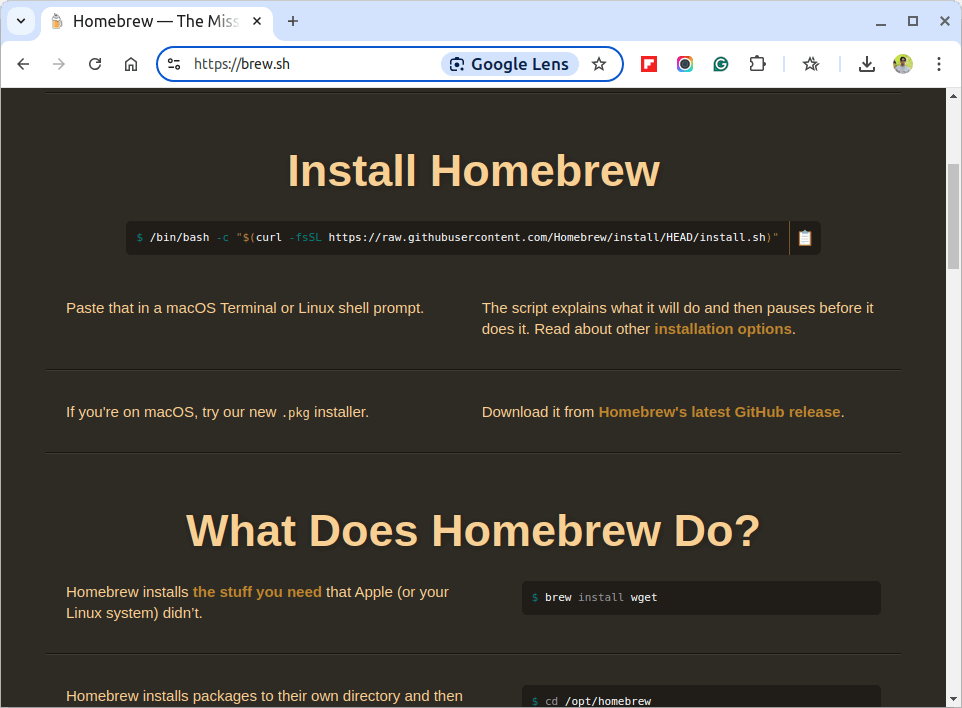
pip
pip is the default package manager for Python that helps you install and manage Python libraries and dependencies.
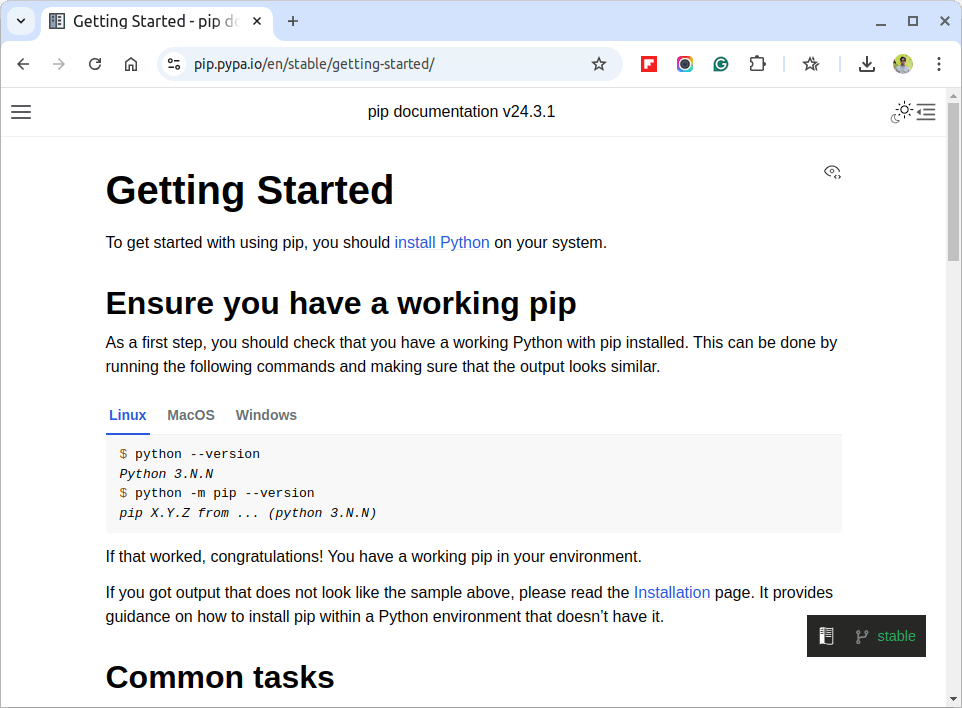
Using package managers can save you a lot of time by managing all the dependencies your app needs and making sure they are up to date.
4. Containerization and Virtualization
Containers allow developers to package an app and its dependencies together, making it easier to run the app in different environments, such as development, testing, and production. Virtualization tools are also helpful for testing your app in different environments.
Docker
Docker is a tool that enables developers to package applications and their dependencies into containers, and these containers can run consistently on any machine, whether on your local computer, a cloud server, or in a production environment.
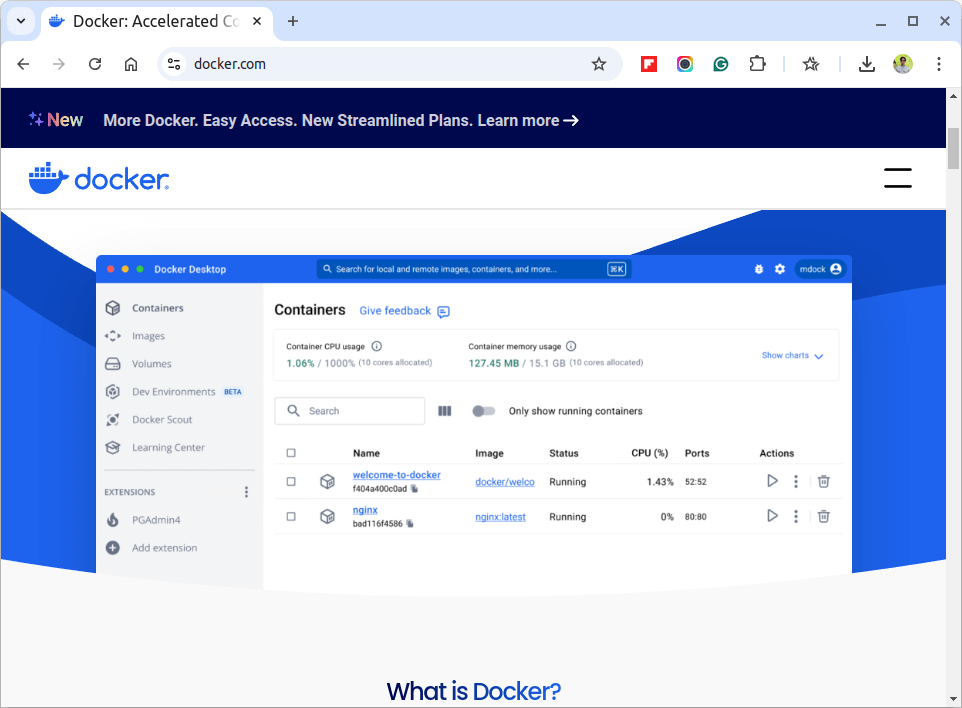
Kubernetes
Kubernetes is a system for automating the deployment, scaling, and management of containerized applications, which is ideal for larger projects where you need to manage multiple containers.
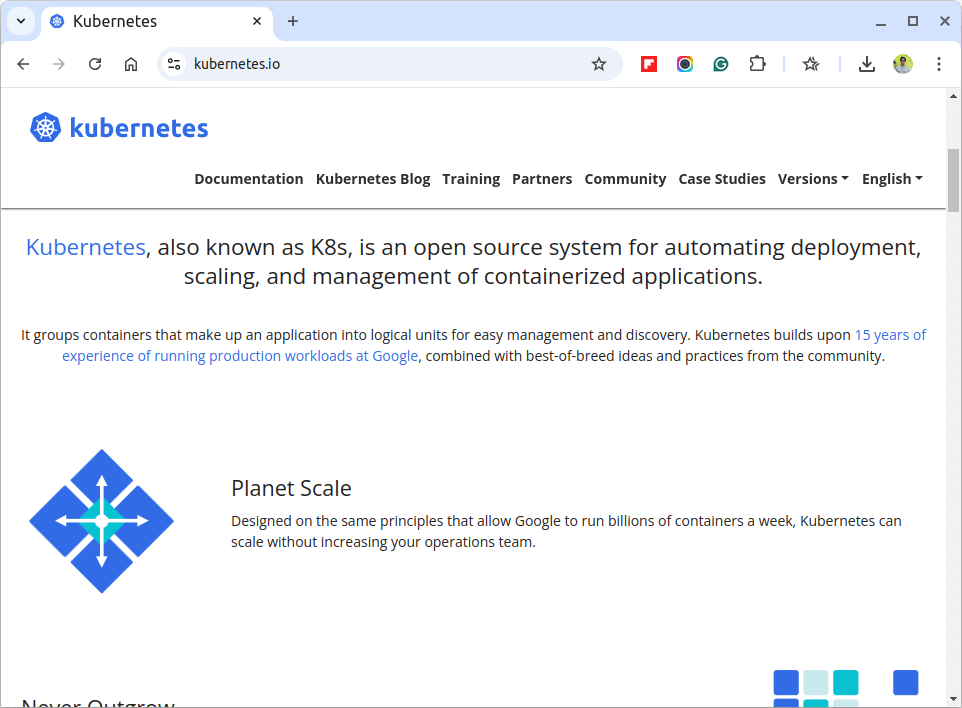
Vagrant
Vagrant is a tool for building and maintaining virtual machine environments, it allows you to create a virtual machine with the required software and dependencies for your app, making it easier to share development environments across teams.
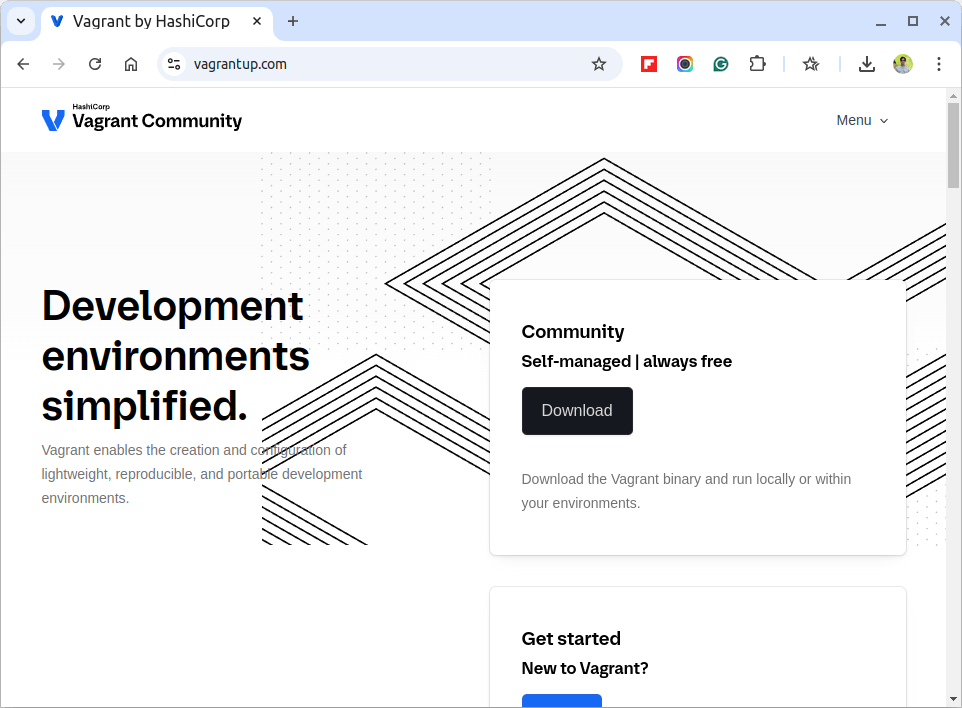
Using Docker and Kubernetes ensures your app will run smoothly in different environments, reducing “works on my machine” issues.
5. Database Management Tools
Most modern apps need to interact with a database to store and retrieve data. Whether you’re using a relational database like MySQL or a NoSQL database like MongoDB, managing and interacting with these databases is an essential part of app development.
MySQL Workbench
MySQL Workbench is a graphical tool for managing MySQL databases, it offers an easy-to-use interface for writing queries, creating tables, and managing your database.
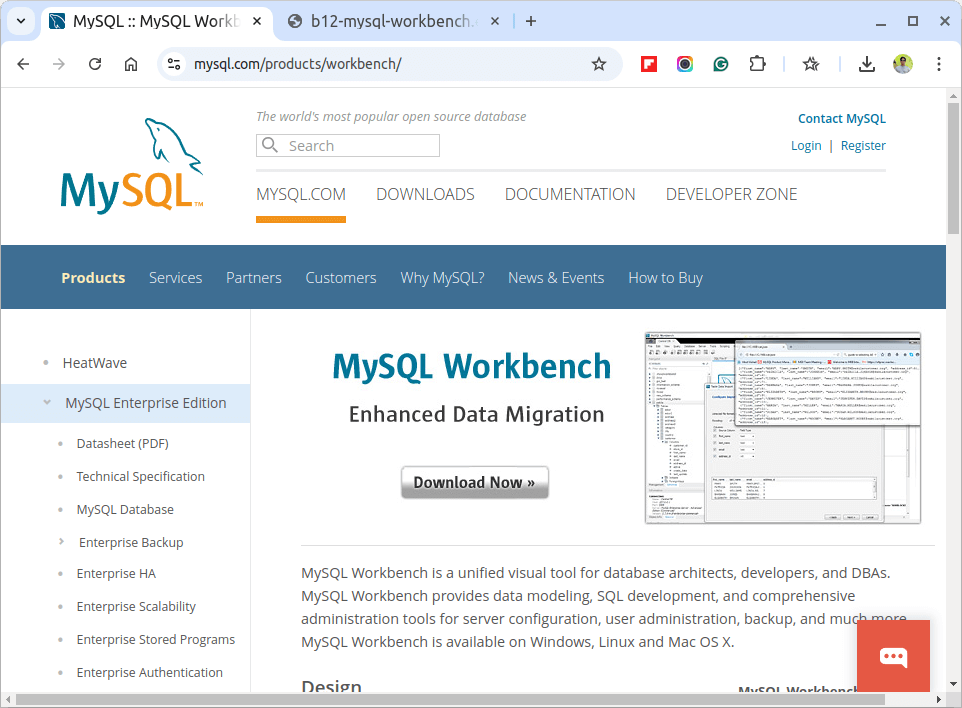
pgAdmin
pgAdmin is a management tool for PostgreSQL databases, offering a rich set of features for interacting with your database, writing queries, and performing administrative tasks.
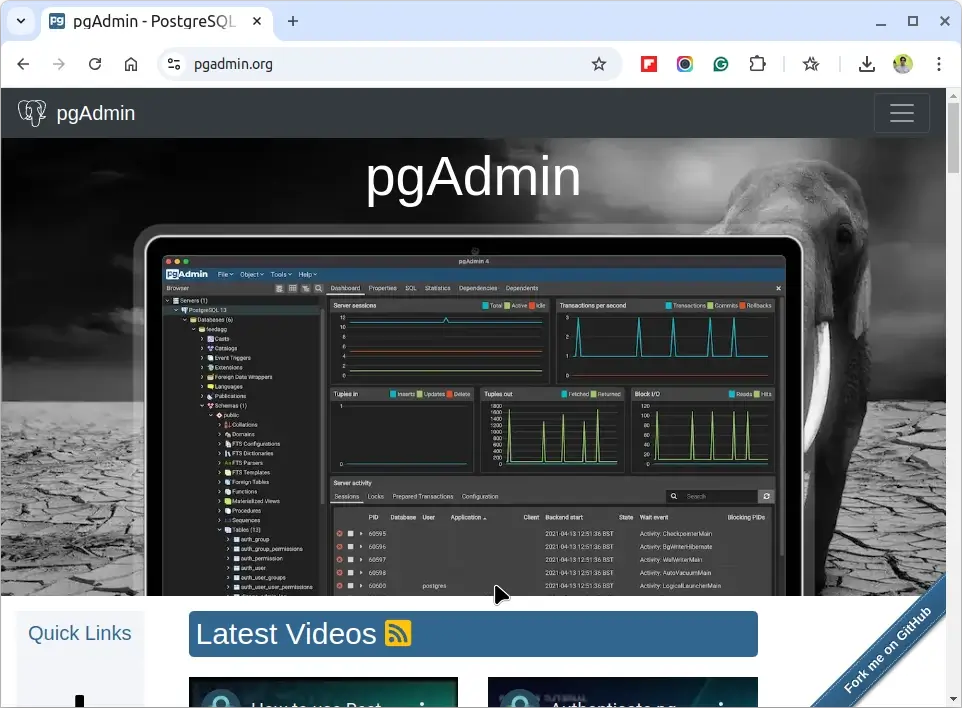
MongoDB Compass
MongoDB Compass is a GUI for MongoDB that allows you to visualize your data, run queries, and interact with your NoSQL database.
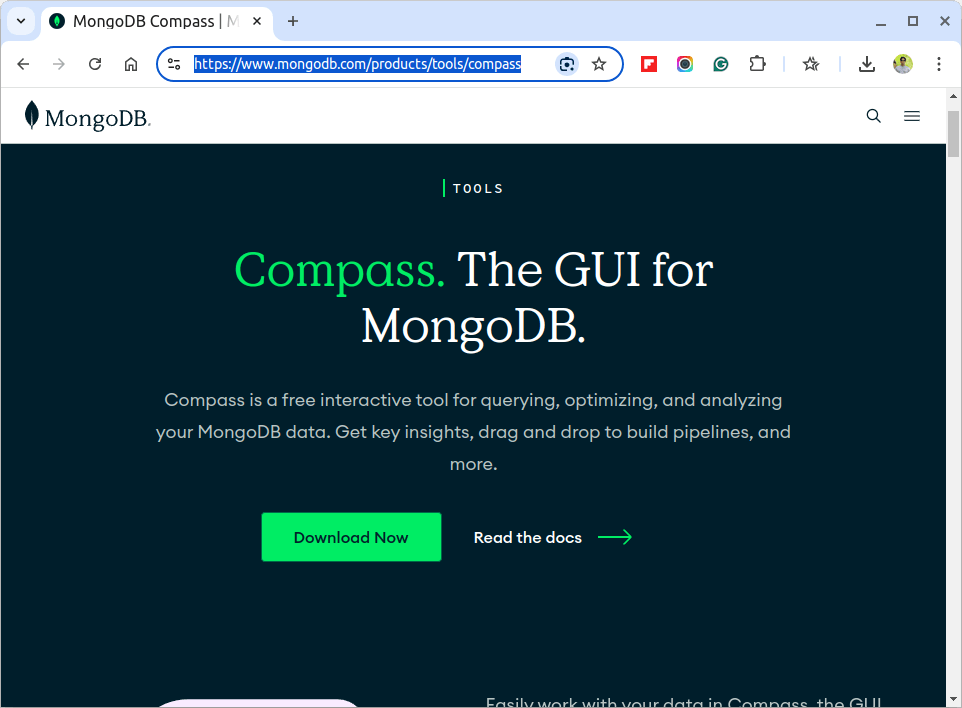
DBeaver
DBeaver is a universal database management tool that supports multiple databases, including MySQL, PostgreSQL, SQLite, and others.
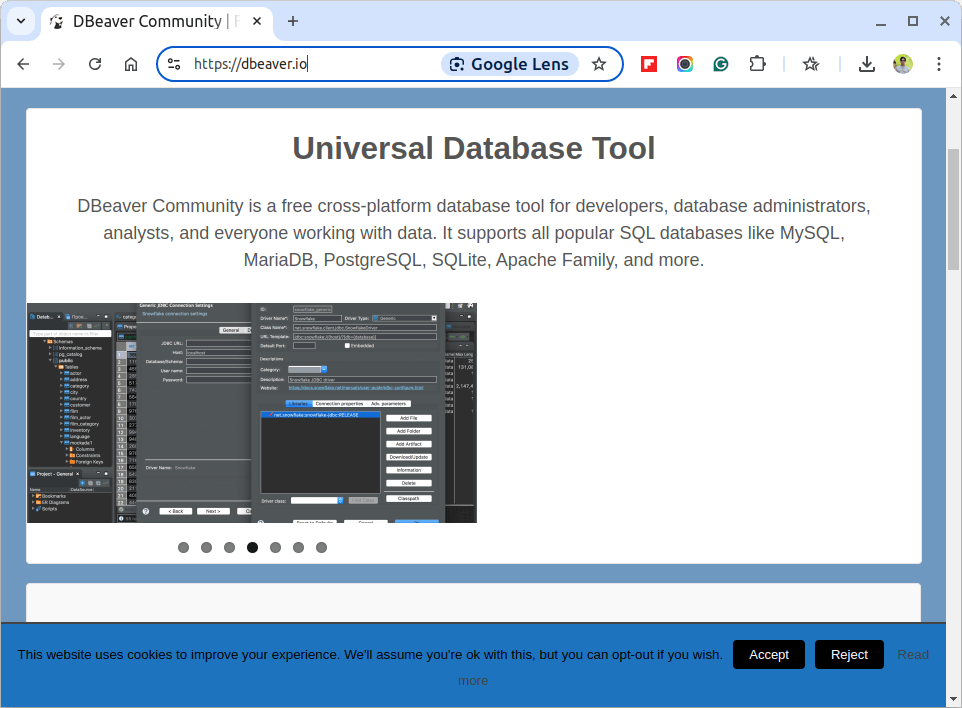
Having a good database management tool helps you efficiently interact with and manage your app’s database.
6. API Development Tools
Modern apps often rely on APIs (Application Programming Interfaces) to interact with other services or allow third-party apps to interact with your app. API development tools help you design, test, and manage APIs efficiently.
Postman
Postman is a popular tool for testing APIs, which allows you to send HTTP requests, view responses, and automate API tests. Postman is especially helpful during the development and testing phase of your app.
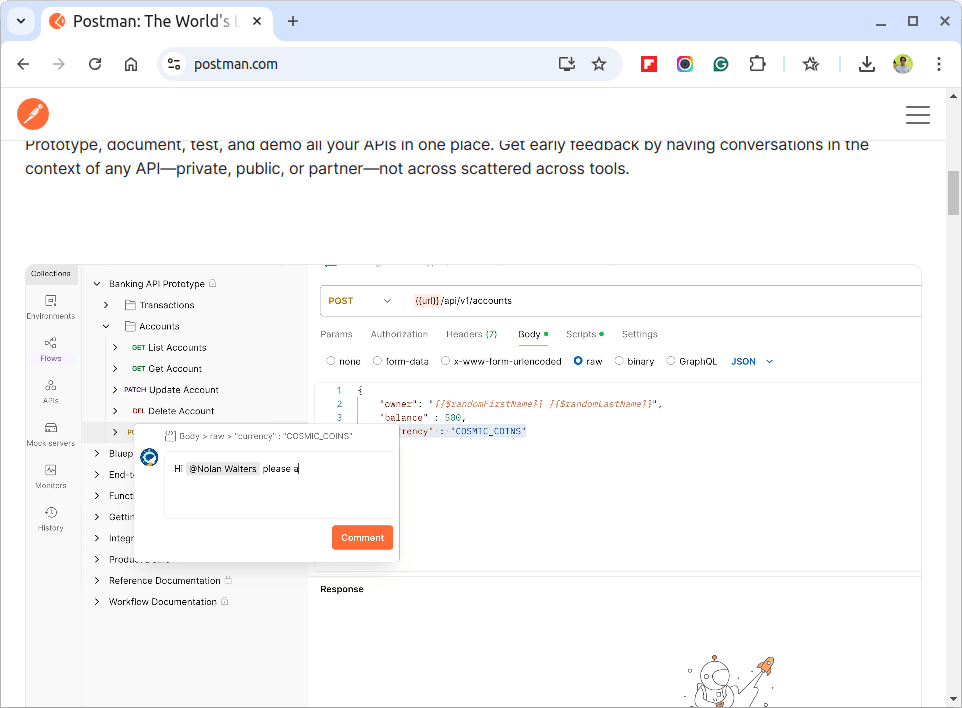
Swagger/OpenAPI
Swagger/OpenAPI is a framework for designing, building, and documenting RESTful APIs. Swagger can generate interactive API documentation that makes it easier for other developers to understand and use your API.
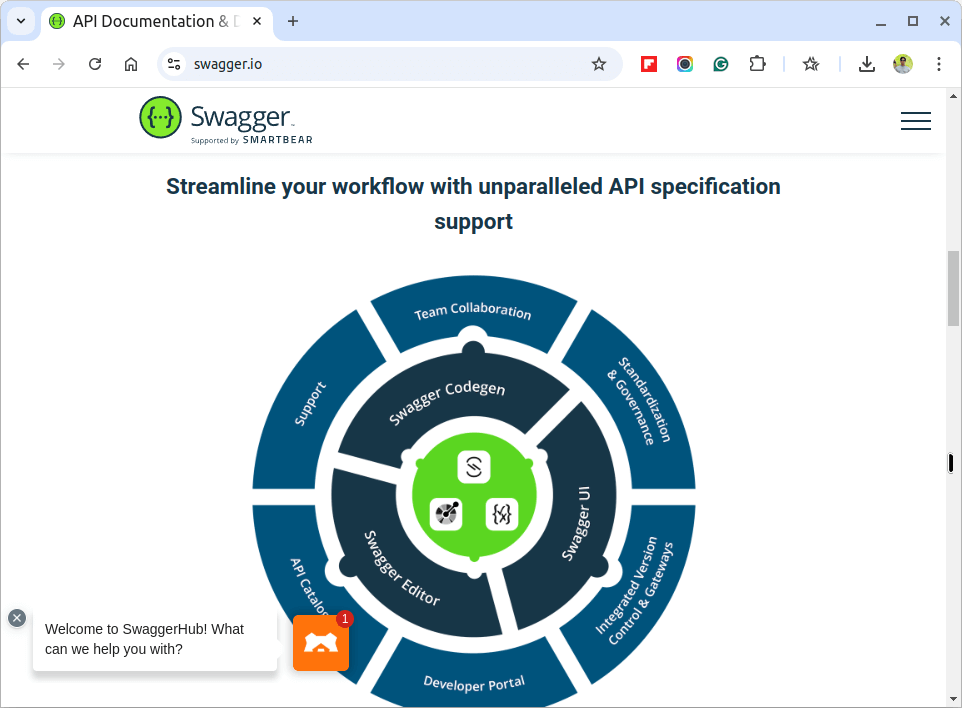
Insomnia
Insomnia is another API testing tool similar to Postman, but with a focus on simplicity and ease of use. It’s great for developers who want a lightweight tool to test APIs without too many distractions.
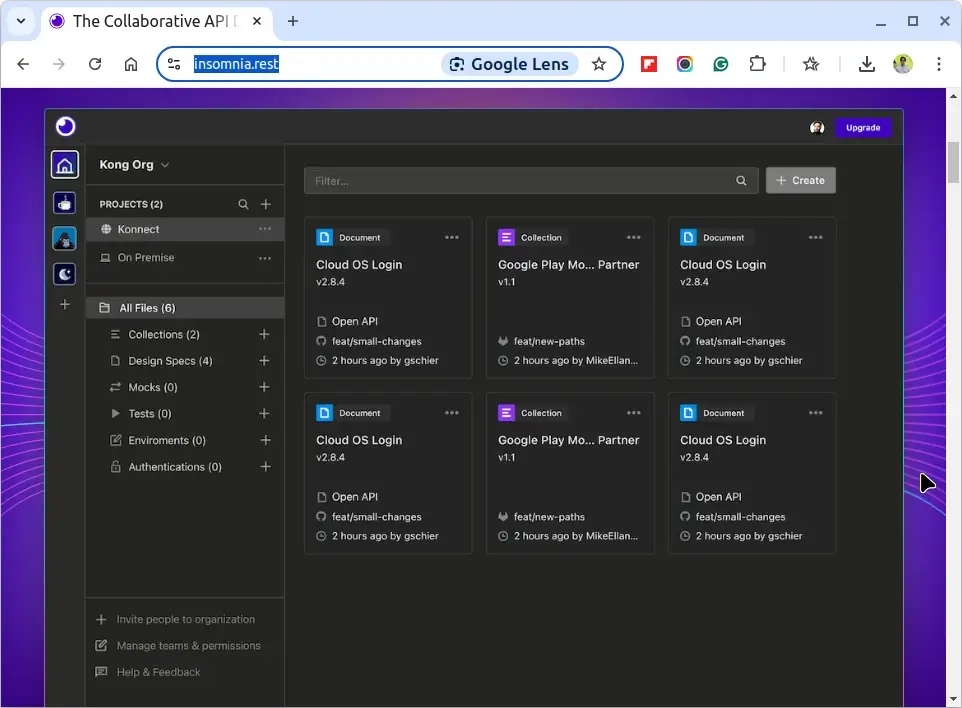
Using API development tools can make it easier to test and debug your app’s integration with external services.
7. Testing Tools
Testing is a crucial step in building modern apps, which ensures that your app works correctly and provides a good user experience. Whether you’re testing individual pieces of code (unit testing) or the entire app (end-to-end testing), the right tools are essential.
JUnit
JUnit is a framework for writing and running unit tests in Java. It’s widely used in the Java development community.
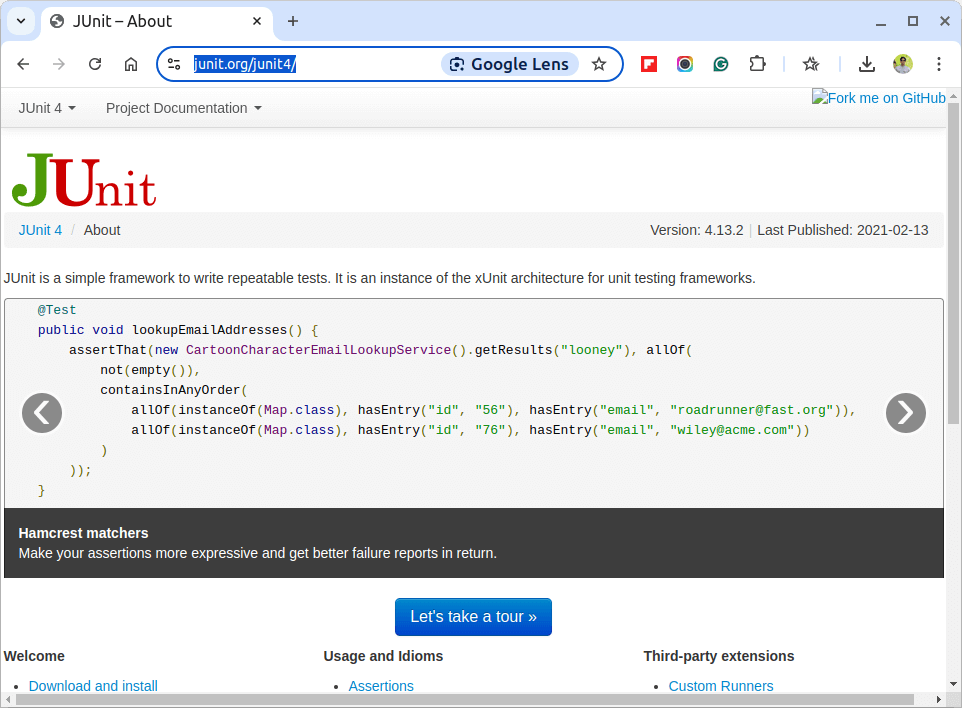
Mocha
Mocha is a JavaScript testing framework that runs in Node.js
and in the browser, and helps you write tests for your app’s behavior.
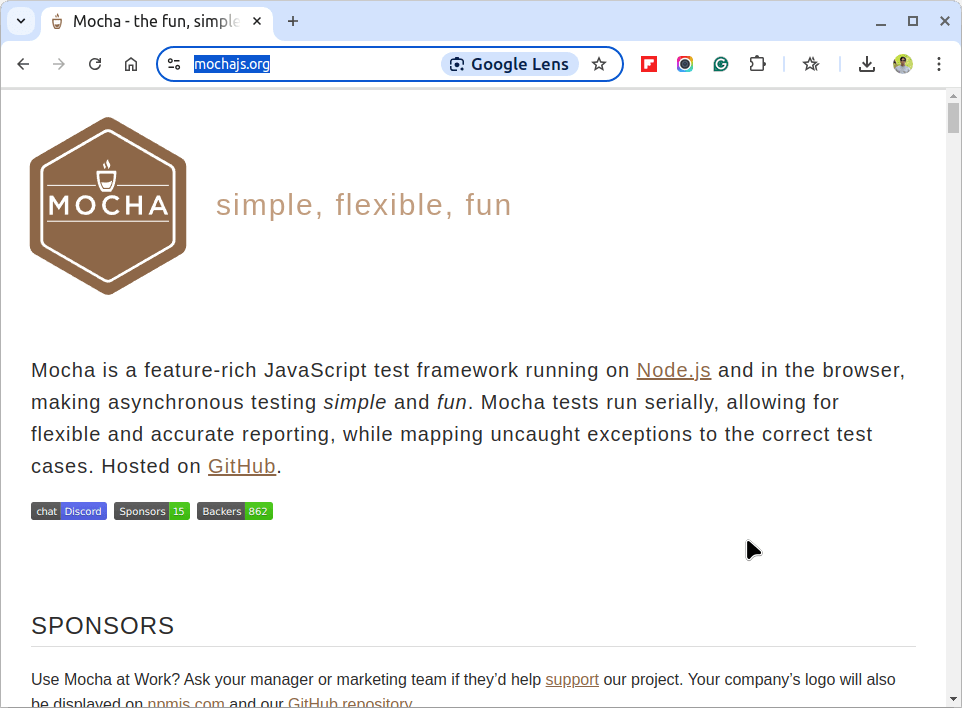
Selenium
Selenium is a tool for automating web browsers, allowing you to perform end-to-end testing of your web app’s UI.
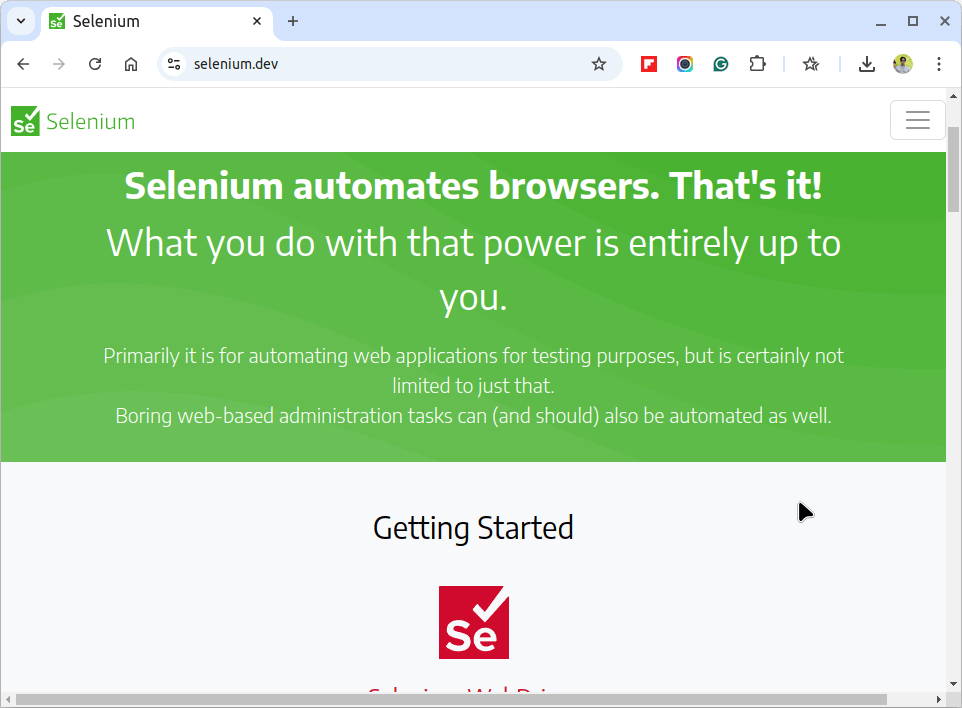
Jest
Jest is a testing framework for JavaScript that works well with React and other JavaScript frameworks. Jest offers fast and reliable tests with great debugging features.
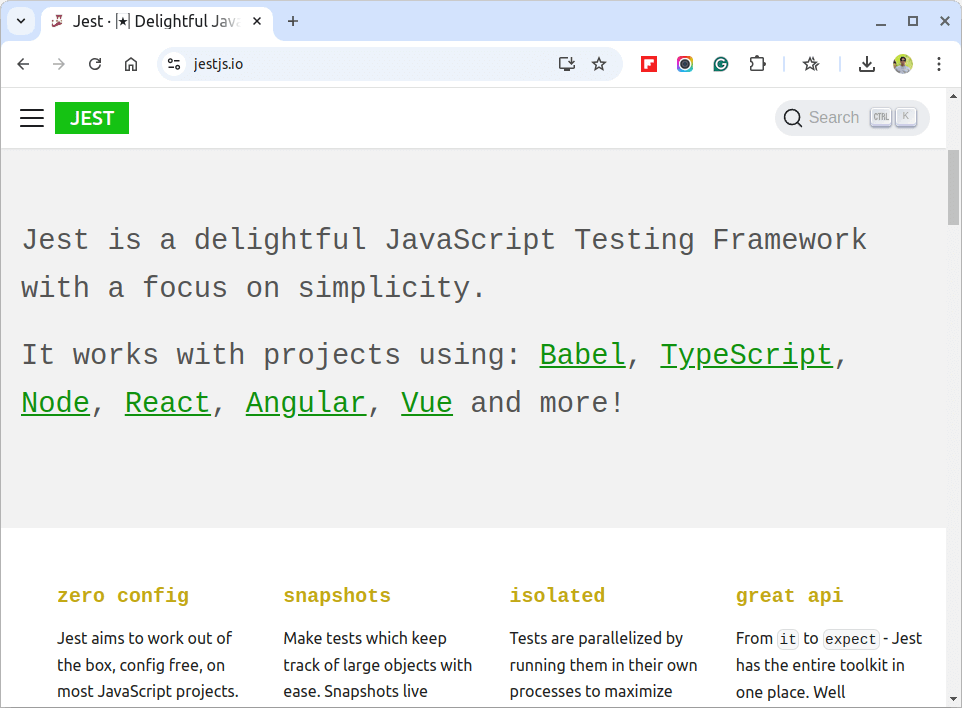
Good testing tools help you identify bugs early, improve the quality of your app, and ensure that it works as expected.
Continuous Integration and Continuous Deployment (CI/CD) Tools
CI/CD is a modern practice that involves automating the process of testing, building, and deploying your app. CI/CD tools help you ensure that your app is always in a deployable state and can be released to production quickly and reliably.
Jenkins
Jenkins is a popular open-source automation server that allows you to automate building, testing, and deploying your app, it integrates with many version control systems and other tools.
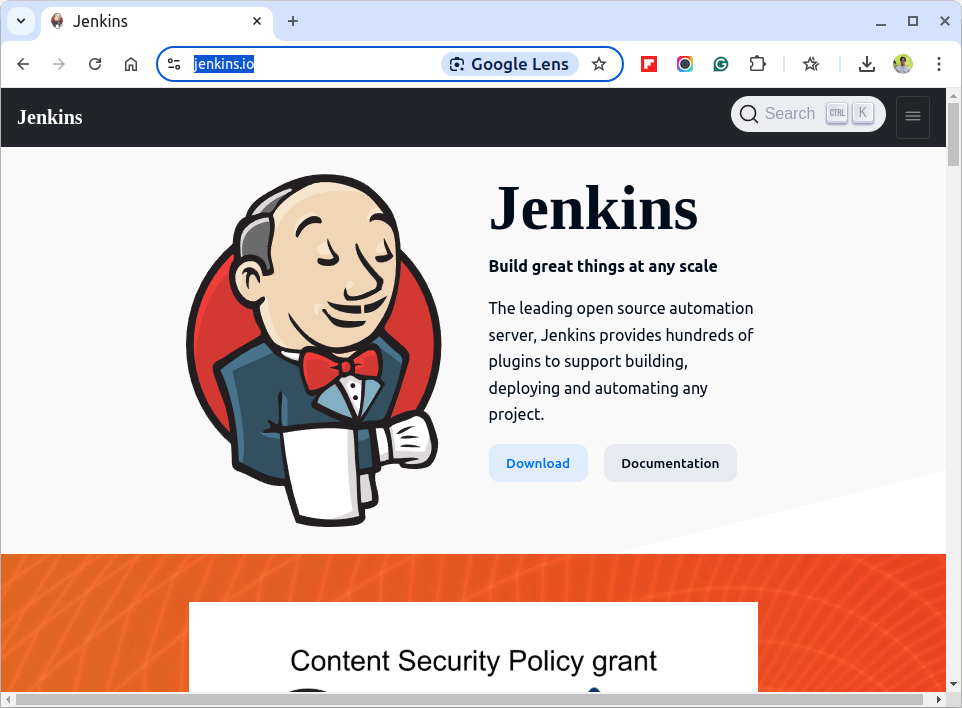
Travis CI
Travis CI is a cloud-based CI/CD service that integrates easily with GitHub and automates the process of testing and deploying your app.
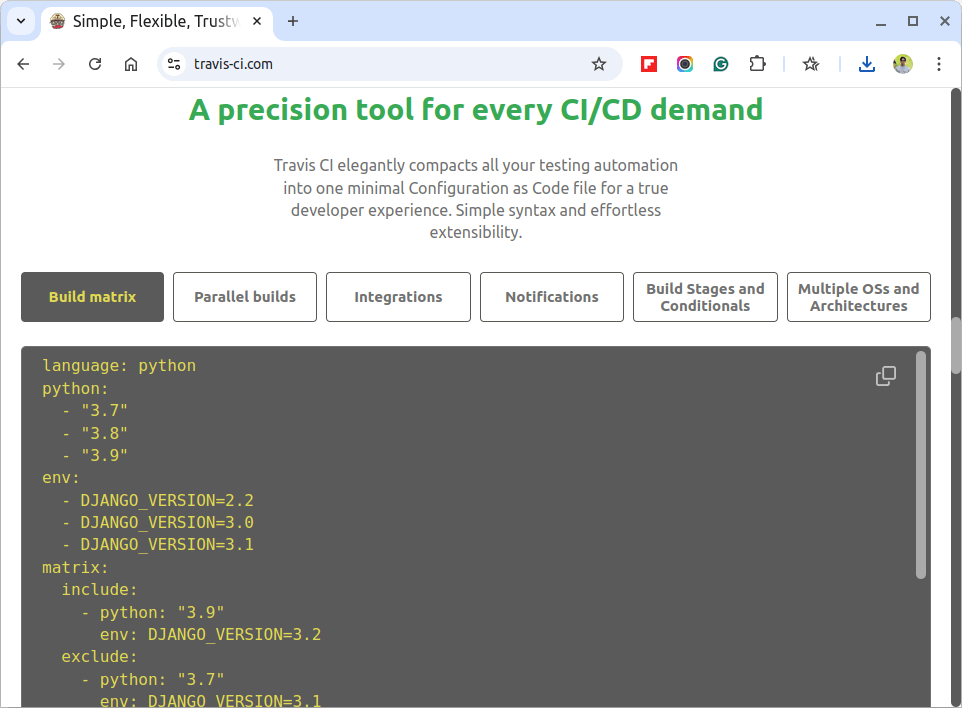
CircleCI
CircleCI is a fast, cloud-based CI/CD tool that integrates with GitHub, Bitbucket, and GitLab, and helps automate the testing and deployment of your app.
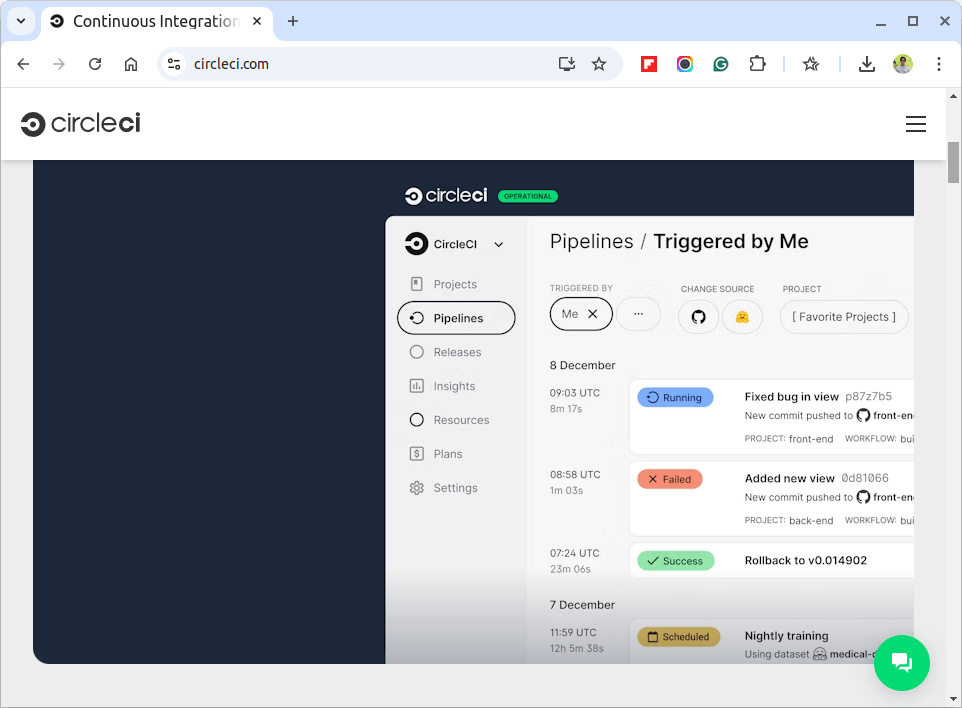
GitLab CI/CD
GitLab CI/CD offers built-in CI/CD features, allowing you to manage the entire software development lifecycle from code to deployment in one platform.
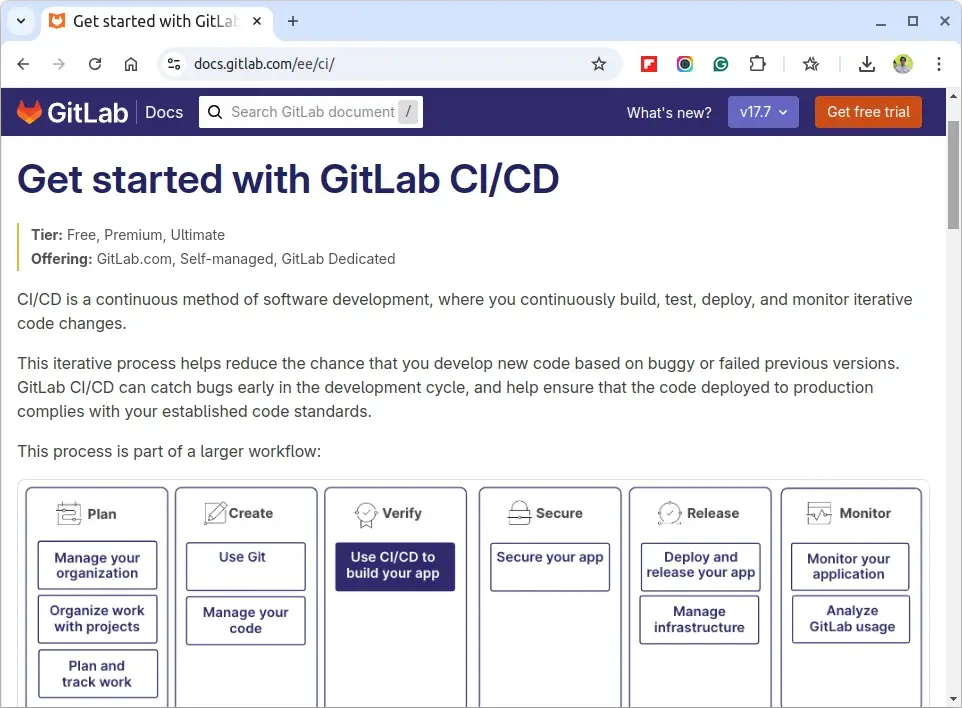
CI/CD tools help automate the repetitive tasks of building, testing, and deploying, saving developers a lot of time and reducing the chances of human error.
9. Cloud Platforms and Hosting Services
For modern apps, hosting them in the cloud is often the best option, as cloud platforms provide scalable infrastructure, security, and high availability for your app.
Amazon Web Services (AWS)
Amazon Web Services (AWS) is a comprehensive cloud platform offering a wide range of services, including computing, storage, databases, machine learning, and more. AWS is ideal for large-scale apps with high traffic.
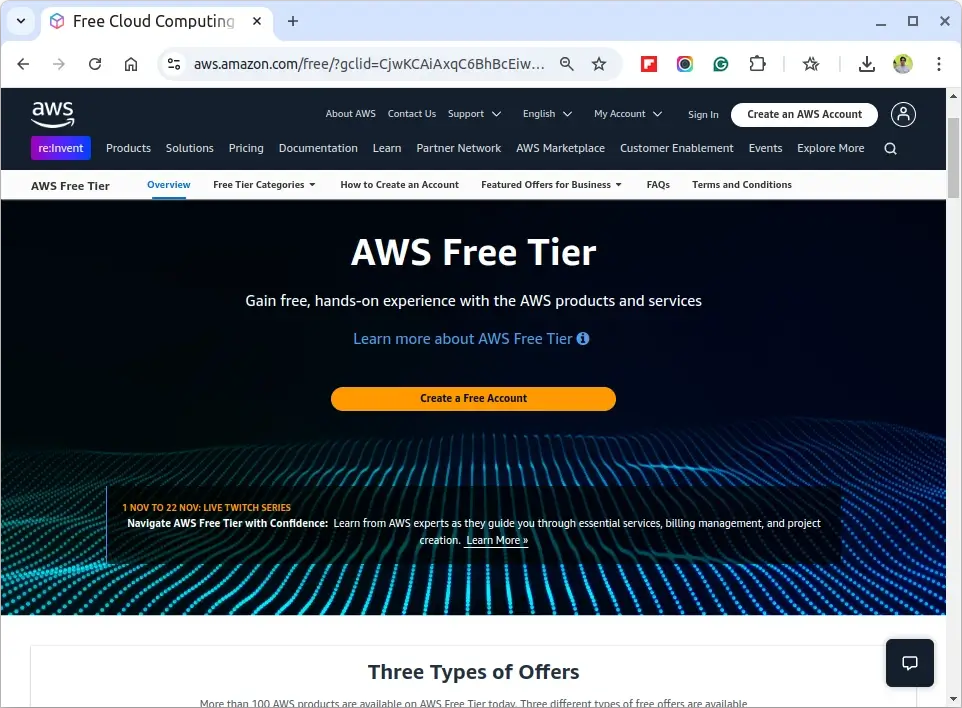
Microsoft Azure
Microsoft Azure is a cloud platform offering various services, including hosting, storage, AI, and databases, which is a popular choice for enterprises and developers building apps on Microsoft technologies.
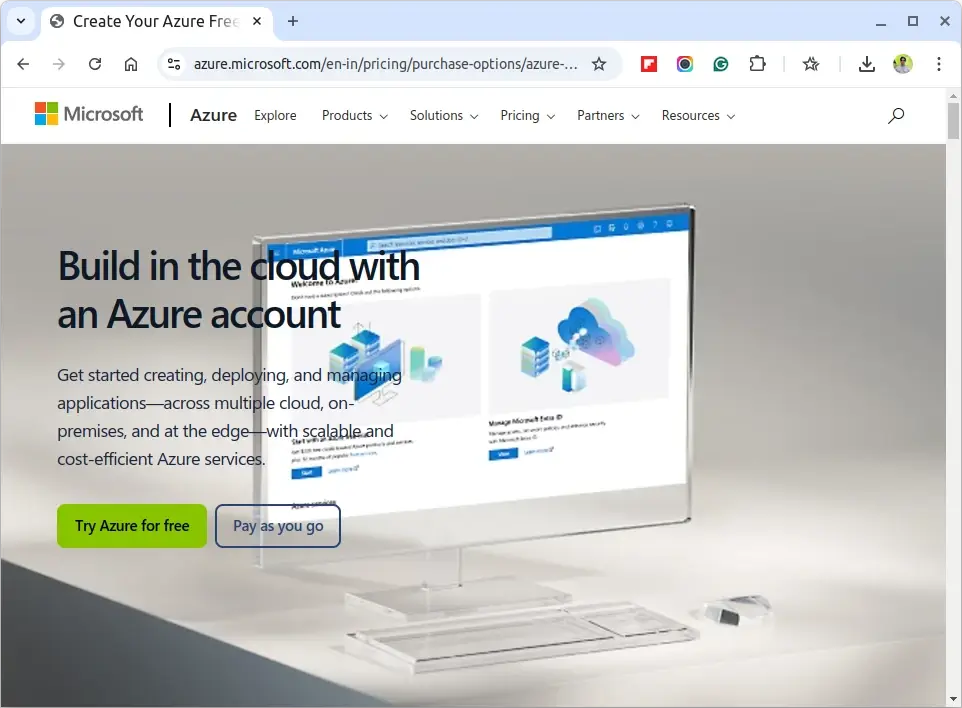
Google Cloud Platform (GCP)
Google Cloud Platform (GCP) offers tools for building, deploying, and scaling applications. GCP is especially popular for apps that rely on machine learning and big data.
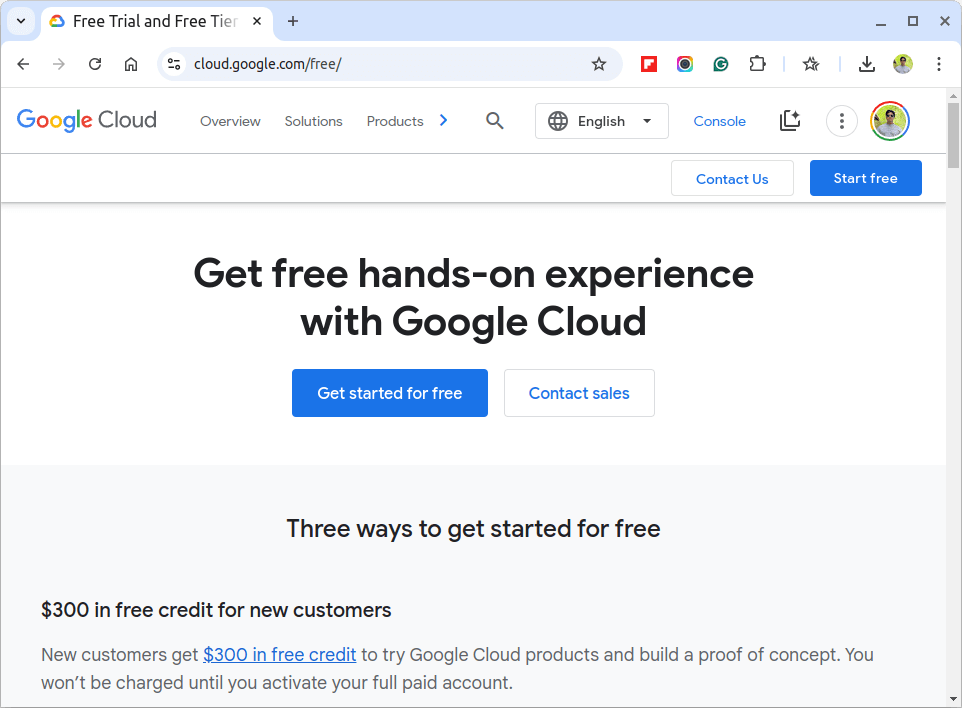
Heroku
Heroku is a platform-as-a-service (PaaS) for building, running, and scaling apps, which is great for smaller apps or when you need a quick and easy way to deploy your app.
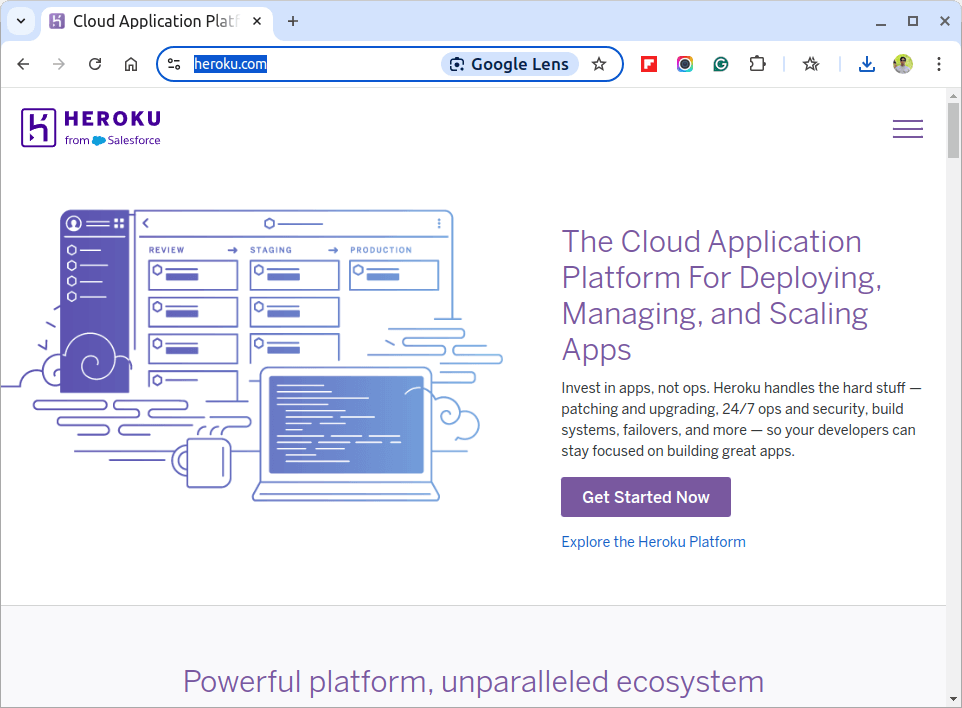
Cloud platforms provide the infrastructure your app needs to run in a scalable, secure, and cost-effective manner.
Conclusion
Building modern apps requires a combination of the right tools to handle different aspects of the development process. Whether you’re writing code, managing dependencies, testing your app, or deploying it to the cloud, having the right tools can make a huge difference in your productivity and the quality of your app.
By using the tools mentioned above, you’ll be well-equipped to build, test, and deploy modern apps efficiently. Happy coding!
Thank you for putting this list together. This is really helpful.
Shouldn’t you clarify that this is all about web apps and not so much about desktop or mobile apps?
I’ve encountered this behavior a number of times already. It’s as if “development” these days just means “development of apps for the web“, like tools for the backend, frontend, cloud, etc.
It’s disappointing for those of us working on local, non-cloud applications.
@Grokker,
You’re absolutely right!
The focus has shifted heavily towards web apps, and it can be frustrating for those of us still working on local or non-cloud applications. It would be great to see more attention given to a broader range of development types.